As default, to keep the Editors and Approvers to be notified about the changes in content, Sitecore provides us the “Email action” under “/sitecore/templates/System/Workflow” to support to send the email to recipients.

However, in some cases, we want Editors to have the ability to select specific Approver to review/approve their content instead of sending email to all pre-configured Approvers and allow Approver to send the approval result back to the Editor who submits previously. This will have some benefit:
- Avoid sending a lot of emails to Approvers in Sitecore or outside of Sitecore
- The interaction between Editors and Approvers will be easier
- Content is managed more effectively by grouping and assigning Approvers to groups
In this blog, I will share an approach to accomplish this, the idea is:
- Create a custom “Droplist” to display all Approvers for Editors to select in the “Comment Template”
- Create a “Comment Template” inherits from “/sitecore/templates/System/Workflow/Standard Comment Template” to add the Approvers field from #1
- Create a custom action to read the information in the “Common Template” to send the email
- Leverage “Sitecore WorkflowProvider” to get the Editor who submits the request and send an email back to Editor when Approver approves or rejects.
Step 1. Go to “Core” database and under “/sitecore/system/Field types/List Types” create a “Publisher Droplist” and update the value of “Control” field to “customcontrol:PublisherDroplist”

Create the Sitecore Patch for this custom field as below. For the simple code behind, you can download here (you can download and edit for your own 😊)
<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/" xmlns:set="http://www.sitecore.net/xmlconfig/set/">
<sitecore>
<controlSources>
<source mode="on" namespace="DemoSite.Foundation.SitecoreExtensions.Controls" assembly="DemoSite.Foundation.SitecoreExtensions" prefix="customcontrol" />
</controlSources>
</sitecore>
</configuration>
Step 2. Create custom “Comment Template” inherits “/sitecore/templates/System/Workflow/Standard Comment Template” and add the field “Publisher” with the field type “Publisher Droplist”

In the “Submit” command under “Draft” stage, select the custom “Comment Template” above for the field “Comment Template”

Step 3. Create custom actions to send the email to Approver from Editor and vice versa.
public class SubmitAction
{
public void Process(WorkflowPipelineArgs args)
{
Assert.ArgumentNotNull(args, "args");
ProcessorItem processorItem = args.ProcessorItem;
if (processorItem == null)
{
return;
}
// Stage Item
Item innerItem = processorItem.InnerItem;
// Workflow Item (Content Item)
Item workflowItem = args.DataItem;
// args.CommentFields: this is a "StringDictionary" we can get values of fields "Comments" and "Publisher"
// args.CommentFields["Comments"]
// args.CommentFields["Publisher"]
if (new WorkflowHelper().SendEmail(innerItem, workflowItem, args.CommentFields, "Submit"))
{
SheerResponse.Alert("The notification has been sent.", Array.Empty<string>());
}
else
{
SheerResponse.Alert("The notification could not be sent.", Array.Empty<string>());
}
}
}
public class ApproveAction
{
public void Process(WorkflowPipelineArgs args)
{
Assert.ArgumentNotNull(args, "args");
ProcessorItem processorItem = args.ProcessorItem;
if (processorItem == null)
{
return;
}
// Stage Item
Item innerItem = processorItem.InnerItem;
// Workflow Item (Content Item)
Item workflowItem = args.DataItem;
// args.CommentFields: this is a "StringDictionary" we can get the value of field "Comments"
// args.CommentFields["Comments"]
if (new WorkflowHelper().SendEmail(innerItem, workflowItem, args.CommentFields, "Approve"))
{
SheerResponse.Alert("The notification has been sent.", Array.Empty<string>());
}
else
{
SheerResponse.Alert("The notification could not be sent.", Array.Empty<string>());
}
}
}
Update to the Email Action for “Draft” and “Awaiting Approval” stages:


Step 4. To get the exact Editor who submits the request previously, we use “WorkflowProvider” to get the latest historical change of the item:
public string GetSubmittingUserEmail(Item workflowItem)
{
string submittingUserEmail = string.Empty;
try
{
var contentWorkflow = workflowItem.Database.WorkflowProvider.GetWorkflow(workflowItem);
// Get historical data for the workflow item
var contentHistory = contentWorkflow.GetHistory(workflowItem);
if(contentHistory != null && contentHistory.Any())
{
// Get the latest change from the Editor
var submittingHistory = contentHistory.OrderByDescending(o => o.Date).FirstOrDefault(o => o.NewState.ToString() == "[The ID of your 'Awaiting Approval' stage item]");
if(submittingHistory != null)
{
string lastUser = submittingHistory.User;
// Get Editor user
User submittingUser = GetUserByUserName(lastUser);
submittingUserEmail = submittingUser.Profile?.Email ?? string.Empty;
}
}
}
catch (Exception ex)
{
Log.Error($"[WORKFLOW] - GetSubmittingUserEmail ERROR: {ex.Message}", ex, this);
}
return submittingUserEmail;
}
public User GetUserByUserName(string userName)
{
if (User.Exists(userName))
return User.FromName(userName, false);
return null;
}
Deploy the code base and let’s try it.
Login to Sitecore using Editor account and create a “Testing Page”:

Submit the change, the comment dialog will appear, allows Editor to select the Approver as blow. Click “OK”, an email will be sent to “approveruser1”


Logout and login to Sitecore using the account “approveruser1” and approve the change.

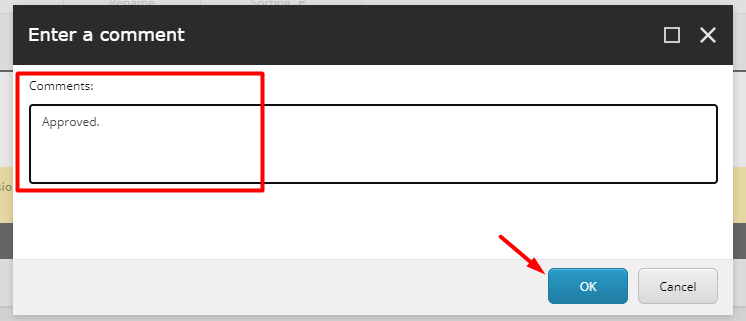
An email will be sent back to the Editor

To view the historical changes of “Testing Page”, on the ribbon, navigate to “Review” -> chunks “Workflow” -> click on “History”:
Historical changes before submitting request by Editor:

Historical changes after approved by Approver:

References:
- https://doc.sitecore.com/en/users/90/sitecore-experience-platform/workflows-and-the-workbox.html
- https://community.sitecore.net/technical_blogs/b/sitecore_development_team/posts/customizable-workflow-comments
- https://iarpitkatiyar.com/2017/02/08/custom-language-selector-in-sitecore/
Got issues?
If you get issues or would like to discuss more, please leave a message or drop me an email at [email protected].
Thanks for reading and happy Sitecore coding!
Related Articles: